How do you create a traceback in Python?
Table of Contents
How do you create a traceback in Python?
There’s no documented way to create traceback objects. None of the functions in the traceback module create them. You can of course access the type as types. TracebackType , but if you call its constructor you just get a TypeError: cannot create ‘traceback’ instances .
How do I fix traceback error in Python?
Because you enter a string, Python treats it as a name and tries to evaluate it. If there is no variable defined with that name you will get a NameError exception. To fix the problem, in Python 2, you can use raw_input() . This returns the string entered by the user and does not attempt to evaluate it.
How do I read a Python traceback?
How Do You Read a Python Traceback?

- Blue box: The last line of the traceback is the error message line.
- Green box: After the exception name is the error message.
- Yellow box: Further up the traceback are the various function calls moving from bottom to top, most recent to least recent.
How do you trace in Python?
Function in the ‘trace’ module in Python library generates trace of program execution, and annotated statement coverage. It also has functions to list functions called during run by generating caller relationships. Following two Python scripts are used as an example to demonstrate features of trace module.
What is traceback issue?
In Python, A traceback is a report containing the function calls made in your code at a specific point i.e when you get an error it is recommended that you should trace it backward(traceback). Whenever the code gets an exception, the traceback will give the information about what went wrong in the code.
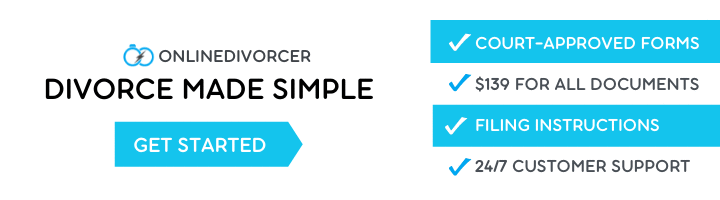
How do I print stack elements in Python?
Below are the steps:
- Push the top element from the given stack into a linked list stack.
- Print the top element of the singly linked list stack.
- Pop the top element from the given primary stack.
- Repeat the above steps in order until the given stack is empty.
What is the trace function in Python?
The trace module allows you to trace program execution, generate annotated statement coverage listings, print caller/callee relationships and list functions executed during a program run. It can be used in another program or from the command line.
What is stack trace in Python?
The Python stack trace is a valuable piece of information that you can use to debug your code. It contains information about the call stack and points out where things have gone wrong. At the end of a stack trace, you can always find the exact exception type and a detailed message of what’s gone wrong.
How do you trace a stack?
Accessing Stack Traces with the Thread Class You can obtain a stack trace from a thread – by calling the getStackTrace method on that Thread instance. This invocation returns an array of StackTraceElement, from which details about stack frames of the thread can be extracted.
What is a ValueError in Python?
Overview. ValueError in Python is raised when a user gives an invalid value to a function but is of a valid argument. It usually occurs in mathematical operations that will require a certain kind of value, even when the value is the correct argument.
What causes EOFError Python?
EOFError is raised when one of the built-in functions input() or raw_input() hits an end-of-file condition (EOF) without reading any data. This error is sometimes experienced while using online IDEs. This occurs when we have asked the user for input but have not provided any input in the input box.
How do I print a stack in reverse order?
Use Recursion Pop the top element from the stack and make a recursive call till the stack is not empty. This will store all the stack elements into function stack in reverse stack. In tail recursion print the elements and restore elements in the originally given array.
How do you reverse a stack?
Solution Steps
- Create a recursive function recur to reverse the stack.
- Pop the top element in each stack of recursion and hold the element in function call Stack until we reach the end of the stack.
- While moving back in the recursion tree, push the held element of each recursion call stack at the bottom of the stack.