How do you find roots of an equation in Python?
Table of Contents
How do you find roots of an equation in Python?
Python Program to Find the Roots of a Quadratic Equation
- Take in the coefficients of the equation and store it in three separate variables.
- Find the value of the discriminant, d.
- Use an if statement to check if the value of the discriminant is greater than 0 or lesser than 0.
How do you find roots in programming?
Program to Find Roots of a Quadratic Equation
- Add Two Complex Numbers by Passing Structure to a Function.
- Find the Largest Number Among Three Numbers.
- Find GCD of two Numbers.
- Check Prime or Armstrong Number Using User-defined Function.
How do you find the quadratic equation in Python?

We have imported the cmath module to perform complex square root. First, we calculate the discriminant and then find the two solutions of the quadratic equation. You can change the value of a , b and c in the above program and test this program.
What does abs () do in Python?
The abs() function returns the absolute value of the specified number.
How do you solve equations in Python?
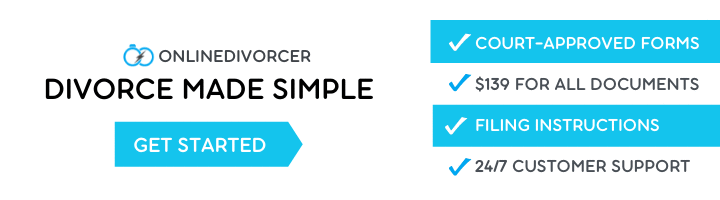
To solve the two equations for the two variables x and y , we’ll use SymPy’s solve() function. The solve() function takes two arguments, a tuple of the equations (eq1, eq2) and a tuple of the variables to solve for (x, y) . The SymPy solution object is a Python dictionary.
How do you program the roots of a quadratic equation?
Design (Algorithm)
- Start.
- Read a, b, c values.
- Compute d = b2 4ac.
- if d > 0 then. r1 = b+ sqrt (d)/(2*a) r2 = b sqrt(d)/(2*a)
- Otherwise if d = 0 then. compute r1 = -b/2a, r2=-b/2a. print r1,r2 values.
- Otherwise if d < 0 then print roots are imaginary.
- Stop.
What is all () in Python?
Python all() Function The all() function returns True if all items in an iterable are true, otherwise it returns False. If the iterable object is empty, the all() function also returns True.
What does POW mean in Python?
x to the power of y
Python pow() Function The pow() function returns the value of x to the power of y (xy). If a third parameter is present, it returns x to the power of y, modulus z.
How do you do square root in Python?
sqrt() function is an inbuilt function in Python programming language that returns the square root of any number. Syntax: math. sqrt(x) Parameter: x is any number such that x>=0 Returns: It returns the square root of the number passed in the parameter.
What is Python solver?
solve(expression) method, we can solve the mathematical equations easily and it will return the roots of the equation that is provided as parameter using sympy. solve() method. Syntax : sympy.solve(expression) Return : Return the roots of the equation.
How do you find the root of an equation?
The roots are calculated using the formula, x = (-b ± √ (b² – 4ac) )/2a. Discriminant is, D = b2 – 4ac. If D > 0, then the equation has two real and distinct roots. If D < 0, the equation has two complex roots.