How do you identify a loop in a linked list?
Table of Contents
How do you identify a loop in a linked list?
The idea is to have two references to the list and move them at different speeds. Move one forward by 1 node and the other by 2 nodes. If the linked list has a loop they will definitely meet. Else either of the two references(or their next ) will become null .
How do you find a loop node in a linked list?
Write a function findFirstLoopNode() that checks whether a given Linked List contains a loop. If the loop is present then it returns point to the first node of the loop. Else it returns NULL.
Is it possible to find a loop in a linked list complexity?

The normal scenario to find the loop in the linked list is to move a pointer once and move other pointer two times. If they meet,there is a loop in the linked list.
What is the optimal way to detect a loop in a doubly linked list?
Method 2 (Better Solution) Let the count be k. Fix one pointer to the head and another to a kth node from the head. Move both pointers at the same pace, they will meet at loop starting node. Get a pointer to the last node of the loop and make next of it as NULL.
Is cycle present in LinkedList?
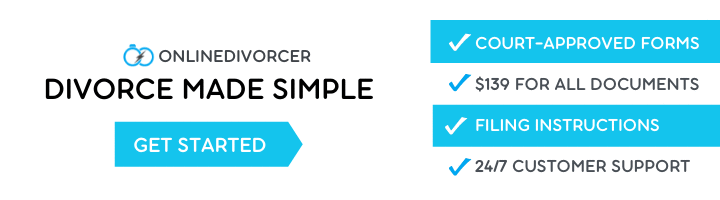
There is a cycle in a linked list if there is some node in the list that can be reached again by continuously following the next pointer.
What is a cycle in a linked list?
A cycle occurs when a node’s next points back to a previous node in the list. The linked list is no longer linear with a beginning and end—instead, it cycles through a loop of nodes.
What is a loop in linked list?
A loop in a linked list is a condition that occurs when the linked list does not have any end. When the loop exists in the linked list, the last pointer does not point to the Null as observed in the singly linked list or doubly linked list and to the head of the linked list observed in the circular linked list.
How do you find the first node in a circular linked list?
Our approach will be simple:
- Firstly, we have to detect the loop in the given linked list.
- In Floyd’s cycle detection algorithm, we initialize 2 pointers, slow and fast.
- Now, after finding the loop node:
- Now, as we have the length of the loop, we will make ptr1 and ptr2 point to the head again.
Can a linked list have cycles?
There is a cycle in a linked list if there is some node in the list that can be reached again by continuously following the next pointer. Internally, pos is used to denote the index of the node that tail’s next pointer is connected to.
Can we find the end of circular linked list?
In a circular Singly linked list, the last node of the list contains a pointer to the first node of the list. We can have circular singly linked list as well as circular doubly linked list. We traverse a circular singly linked list until we reach the same node where we started.
Does linked list allow null values?
LinkedList allow any number of null values while LinkedHashSet also allows maximum one null element.
How do I remove duplicates from LinkedList?
Write a removeDuplicates() function that takes a list and deletes any duplicate nodes from the list. The list is not sorted. For example if the linked list is 12->11->12->21->41->43->21 then removeDuplicates() should convert the list to 12->11->21->41->43.
What is a cycle in linked list?
How do you find the last node in a circular single linked list?
How do you find the last node in a linked list?
The last node of a linked list has the reference pointer as NULL. i.e. node=>next = NULL. To find the last node, we have to iterate the linked till the node=>next != NULL.