Is C++ good for multithreaded?
Table of Contents
Is C++ good for multithreaded?
Starting with C++11 C++ has classes for multithreading support. The class you might be interested in most is std::thread . There are also classes for synchronization like std::mutex .
How do threads communicate with each other in C++?
you can use memory that is shared (provided you synchronize access) you can use queues to pass information from one thread to another (such as with pipes)
How do you create multiple threads in C++?
To start a thread we simply need to create a new thread object and pass the executing code to be called (i.e, a callable object) into the constructor of the object. Once the object is created a new thread is launched which will execute the code specified in callable. After defining callable, pass it to the constructor.

How do you communicate between two threads?
Cooperation (Inter-thread communication) is a mechanism in which a thread is paused running in its critical section and another thread is allowed to enter (or lock) in the same critical section to be executed.It is implemented by following methods of Object class: wait() notify() notifyAll()
How do I transfer data between two threads?
The solution is to use synchronization, such as atomic ints, or locking. The C++ compiler does very impressive optimizations to make your single threaded programs run faster. For example, it may prove that, in a single threaded world, there is no way to see the 1000 value, and simply choose not to print it.
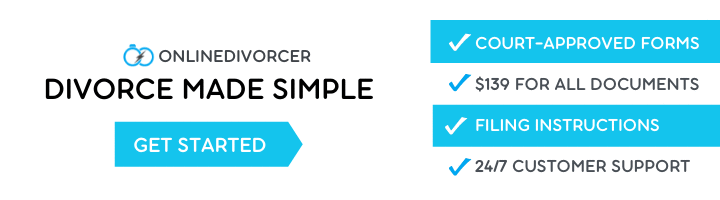
How does multi threading works?
Multithreading is a model of program execution that allows for multiple threads to be created within a process, executing independently but concurrently sharing process resources. Depending on the hardware, threads can run fully parallel if they are distributed to their own CPU core.
How many threads can I run C++?
There is nothing in the C++ standard that limits number of threads. However, OS will certainly have a hard limit. Having too many threads decreases the throughput of your application, so it’s recommended that you use a thread pool.
Do C++ threads run in parallel?
Your threads already run in ‘parallel’ (so to speak), that is the nature of a thread. Your code posted does not have anything that would be ‘parallel’ in nature (i.e. parallel computing of data) but your threads are running concurrently (at the same time, or ‘parallel’ to each).
Why is multithreading so hard?
Multi-threaded programming is probably the most difficult solution to concurrency. It basically is quite a low level abstraction of what the machine actually does. There’s a number of approaches, such as the actor model or (software) transactional memory, that are much easier.
Why is multi threading so hard?
Multithreaded programs seem harder or more complex to write because two or more concurrent threads working incorrectly make a much bigger mess a whole lot faster than a single thread can.
Why is multithreading slow?
Every thread needs some overhead and system resources, so it also slows down performance. Another problem is the so called “thread explosion” when MORE thread are created than cores are on the system. And some waiting threads for the end of other threads is the worst idea for multi threading.
Can two threads in a process communicate?
Threads of the same process can communicate with each other through synchronization primitives like locks and semaphores, events like wait and notify, or through shared memory. The following illustration shows how a process (single-threaded) and a multi-threaded process share memory.
Can multiple threads read the same memory?
Not only are different cores allowed to read from the same block of memory, they’re allowed to write at the same time too. If it’s “safe” or not, that’s an entirely different story.
What is multithreading CPP?
Advertisements. Multithreading is a specialized form of multitasking and a multitasking is the feature that allows your computer to run two or more programs concurrently. In general, there are two types of multitasking: process-based and thread-based.